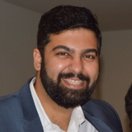
5/20/2023
My latest article - What is Exploratory Testing? Learn with a real world example
Unit Testing
Software testing is essential to ensuring that applications run smoothly and reliably, but did you know that the secret sauce to functional testing success lies in unit testing?
In this article, we'll explore the ins and outs of unit testing, its significance in functional testing, and how it contributes to your software's overall quality and maintainability.
Unit testing is a method for evaluating software that targets individual code units or components in isolation. It aims to validate that each software unit performs as expected, helping developers identify and fix potential defects before they become more significant problems.
Objectives of unit testing
The primary objectives of unit testing are to:
Towards the end of this article, you will also find some frequently asked questions that any new tester has in software engineering.
Importance of Unit Testing
Unit testing offers numerous benefits that contribute to the overall success of functional testing. Let's dive into a few key advantages:
Unit testing aids in the early detection of flaws in software development, saving valuable time and resources.
By testing each unit independently, developers can quickly pinpoint the root cause of an issue and fix it before it snowballs into a bigger problem.
As they say, a stitch in time saves nine (or, in this case, maybe even ninety-nine!).
Unit tests act as a safety net, ensuring that your code meets the desired level of quality.
Well-written tests can also serve as a form of documentation, clearly specifying the expected behavior of individual units.
Moreover, unit tests encourage developers to write clean, modular code, making it easier to understand, maintain, and troubleshoot.
Code refactoring is a natural part of software development as requirements and technologies evolve.
Unit tests provide a safety net that ensures your code remains functional even after changes or updates.
With a solid suite of unit tests, developers can confidently refactor their code without worrying about breaking the application, like a safety harness that lets you dance on the edge of a cliff without fear.
Unit tests provide an additional layer of documentation for the code, making it easier for team members to understand each other's work.
This fosters better collaboration and communication within the team, ensuring everyone stays on the same page as they work together to create a high-quality application.
Plus, it saves everyone from the dreaded game of "Who broke the build?"
Unit Testing Principles
In order to get the most out of unit testing, following some guiding principles is essential. Here are some of the key principles to keep in mind:
Unit tests should be concise and focused on testing a specific aspect of a unit's behavior.
This makes the tests easier to understand and maintain and helps you quickly identify the cause of a test failure.
Remember, a small test is a happy test!
When writing unit tests, testing one functionality at a time is crucial. This ensures that each test is independent and easy to understand.
If a test fails, you'll know exactly which function is not working as expected, making it easier to pinpoint the root cause of an issue.
Give your tests descriptive names that indicate what they are testing.
This makes it easier for others (and future you) to understand each test's purpose and what it should be verifying.
Consider this a small investment in your future sanity.
Unit tests should be self-contained and not depend on external factors or the order in which they're executed.
This ensures that your tests are reliable, repeatable and won't lead to unexpected results due to external dependencies.
Keep the surprises for birthday parties, not your test suite.
Leverage testing frameworks and tools that make writing and executing unit tests more accessible.
These tools often provide features such as assertions, test runners, and reporting capabilities that can simplify the unit testing process and help you get the job done efficiently.
Unit tests should be automated and integrated into your build process, ensuring they run every time your code is built.
This helps you catch issues early and maintain a high level of code quality throughout the development process.
Unit Testing Best Practices
To truly master unit testing, it's essential to follow some best practices that can elevate your testing game. Here are a few critical best practices to consider:
Test-driven development (TDD) is an approach where you write the tests before writing the code.
This ensures that you clearly understand the desired functionality and helps you write code that is easy to test. As a result, your code will be more modular, maintainable, and bug-free.
When testing a unit that interacts with external dependencies, it's essential to use mocking and stubbing to isolate the unit under test.
By doing this, you can ensure that your tests are independent of outside influences and remain focused on the functionality you're testing.
Measure code coverage to ensure that your unit tests cover a sufficient percentage of your codebase.
While 100% code coverage may not always be achievable or necessary, aim for a high level of coverage to ensure that your application is thoroughly tested and less prone to issues.
Integrate unit tests into your continuous integration (CI) process. This ensures that your tests are executed automatically whenever new code is committed, helping you maintain a high level of code quality and catch issues early in the development process.
After all, a stitch in time saves... well, you know the drill by now.
Unit Testing Techniques
Various testing techniques can be employed to test individual code units effectively. Each method has its advantages and is suited to different scenarios. Let's dive into some popular unit testing techniques:
Black-box testing focuses on the code's functionality without considering its internal structure. Testers create test cases based on the input-output specifications without knowing the implementation details.
This technique helps ensure the code meets its requirements and is particularly useful for testing user interfaces or APIs.
White-box testing, also known as glass-box or structural testing, focuses on the internal workings of the code. Testers can access the source code and create test cases based on the code's structure, logic, and control flow.
This technique helps test complex algorithms, data structures, and other aspects of the code that are not directly visible to end users.
Grey-box testing combines elements of both black-box and white-box testing.
Testers need to gain more knowledge of the internal structure of the code, which helps them create more effective test cases.
This technique is beneficial for testing integrations and interactions between different software components.
Equivalence partitioning is a testing technique that divides the input domain into partitions of equivalent values.
Test cases are then created for each partition, assuming all values within the partition will behave similarly.
This approach helps reduce the number of test cases while still providing adequate coverage.
Boundary value analysis is a technique that targets the boundaries of the input domain, as these are often the source of errors.
Test cases are created to target the minimum, maximum, and just inside/outside the boundaries, helping to identify edge cases that might cause issues.
Unit Testing Frameworks and Tools
Numerous unit testing frameworks and tools are available, each catering to different programming languages and development environments. Here's an overview of some popular testing frameworks:
JUnit is a widely used and popular testing framework for Java applications.
It provides a rich set of assertions, test runners, and other features that make it easy to create, execute, and manage unit tests.
NUnit is a popular testing framework for C# applications inspired by JUnit. It supports many testing attributes, assertions, and test runners, making it a powerful choice for .NET developers.
Pytest is a robust and adaptable Python testing framework. It boasts a simple yet expressive syntax and supports a variety of modules and extensions for customizing its behavior.
Mocha is a feature-rich JavaScript testing framework that runs on Node.js and the browser. It provides a flexible and expressive API for defining tests and supports numerous assertion libraries, making it a popular choice for JavaScript developers.
When selecting a unit testing framework, it's essential to consider factors such as:
Considering these factors, you can choose the best unit testing framework for your application/project, ensuring a smooth and effective testing experience.
Unit testing is an integral part of the testing process, as it forms the foundation for other testing levels.
By verifying that individual units of code function correctly, unit testing ensures that the application is more stable and less prone to errors when it undergoes higher-level testing, such as integration, system, and acceptance testing.
Unit testing should not be considered a standalone process but a complementary technique that works with other testing levels.
For a truly comprehensive testing strategy, combining unit testing with other testing methods like integration, system, and acceptance testing is essential.
This holistic approach helps identify and resolve issues at every application level, resulting in a more robust and reliable end product.
Challenges and Common Pitfalls in Unit Testing
One common pitfall in unit testing is the need for adequate test coverage.
Insufficient unit testing can lead to undetected issues and reduced code quality.
To avoid this, it's essential to ensure that your unit tests cover all critical paths and scenarios and a representative sample of edge cases.
While testing edge cases is essential, keeping track of the more common scenarios is also important.
Overemphasizing edge cases can lead to an unbalanced testing strategy that neglects most use cases. Remember, balance is key: test edge cases and common scenarios for a well-rounded unit testing approach.
Unit testing primarily focuses on functional correctness, but it's also crucial to consider non-functional aspects such as performance, security, and usability.
Ignoring these aspects can result in suboptimal application performance and increased vulnerability to security threats.
Another common challenge in unit testing is the efficient organization and maintenance of test cases. Poorly organized tests can be challenging to understand, update, and maintain, decreasing test effectiveness.
It is essential to establish a clear structure for your test cases and keep them well-documented and up-to-date.
In conclusion, unit testing is crucial to any functional testing course. It plays an important role in ensuring that individual units of code function correctly and helps lay a strong foundation for higher-level testing.
Incorporating unit testing into your development process can improve code quality, increase collaboration between team members, and create a more reliable end product.
As we've seen, unit testing can make a significant difference in the success of your functional testing efforts.
By understanding the importance of unit testing and implementing it effectively, you can set your projects up for success and create higher-quality applications that meet and exceed user expectations.
Why not give unit testing a try in your next functional testing course? You may be admirably surprised by the outcome!
where is unit testing done?
who are the testers of unit testing?
when should unit testing be performed?
why unit testing is a waste of time?
why automated unit testing?
why mock unit testing?
what unit tests to write?
can unit testing be done manually?
can unit tests use database?
can unit tests depend on each other?
does unit testing speed up development?
is unit testing worth it?
is unit testing verification or validation?