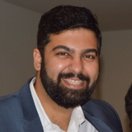
5/11/2024
My latest article - What is Exploratory Testing? Learn with a real world example
Test-Driven Development (TDD): Build Quality Software
Test-Driven Development (TDD) is an Agile-based software development (opens in a new tab) practice that prioritizes the creation of unit test cases before the development of the actual code.
Based on principles of the Agile Manifesto (opens in a new tab) and Extreme programming, (opens in a new tab) TDD enhances the delivery of high-quality software at high speed.
This approach is characterized by a unique cycle: writing failing tests first, followed by developing code to pass these tests, and then refactoring the code.
TDD's philosophy is not just about testing software but about developing a robust framework for software design and functionality.
It offers rapid feedback to developers, enabling them to promptly identify and rectify issues, thereby streamlining the development process.
With its adaptability across various programming languages and its different forms, such as Acceptance TDD (ATDD) and Development TDD (DTDD), TDD has become a versatile and indispensable tool in the developer's arsenal.
Beyond its technical benefits, TDD is lauded for reducing bugs, enhancing code maintainability, and accelerating the pace of development, making it a cornerstone practice in the pursuit of high-quality software development.
Test Driven Development (TDD) is a software development practice that emphasizes the creation of unit test cases before writing the actual code. This approach aligns with the principles of Agile development and is recognized for its efficiency in delivering high-quality software.
TDD is an iterative approach involving programming, unit test creation, and refactoring. Developers create small test cases for every feature based on their understanding.
The core intention here is to modify or write new code only if the tests fail.
This methodology seeks to prevent the duplication of test scripts and produce optimized code that remains resilient in the long term.
To grasp the essence of TDD, let's first understand how it differs from the traditional software development approach.
Traditional Development | Test-Driven Development (TDD) |
---|---|
1. Think about what your code is supposed to do. | 1. Think about what your code is supposed to do. |
2. Write the code to implement the functionality. | 2. Write tests that specify the expected behavior of your code. |
3. Test the code to check if it works as expected. | 3. Write the actual code to fulfill those tests. |
4. Run the tests to verify that the code behaves correctly. |
Table 1: Comparing Traditional Development and Test-Driven Development
In essence, with TDD, you write tests before writing the code itself. This approach offers several advantages and changes the way you develop software.
TDD vs. Traditional Testing
The table below summarizes the primary distinctions between Test Driven Development and traditional testing methodologies:
Aspect | TDD | Traditional Testing |
---|---|---|
Approach | Tests are written before code development. | Tests are performed after code is written. |
Testing Scope | Focuses on testing small units of code one at a time. | Covers the system as a whole, including integration and functional testing. |
Iterative Process | Follows an iterative cycle of test-code-refactor. | Typically follows a linear or waterfall approach. |
Debugging | Errors are caught early in the development phase. | Debugging can be more challenging as errors are found later in the process. |
Documentation | Documentation revolves around test cases and results. | Includes detailed testing plans, environment descriptions, and system specifications. |
Table 2: TDD vs. Traditional Testing: Key Differences
While TDD promotes a disciplined, specification-first approach that can lead to higher quality code and better-architected systems, traditional testing methods provide a comprehensive evaluation of the system after the development phase.
This makes traditional testing especially suitable for larger projects where different aspects of the system are tightly interconnected, and changes in one area might impact several others.
Consider the development of a calculator function. In TDD, you start by writing a test case for the "add" function, followed by the code necessary to make that test pass.
This iterative process continues for other functions such as "subtract," "multiply," and "divide."
Developing an e-commerce website using TDD entails crafting test cases for various features, including product listings, shopping cart functionality, and the checkout process.
These tests ensure the system functions correctly, from adding items to cart to completing the purchase.
We will cover an example of a Fitness app using javascript and JEST framework to showcase the importance of Test driven development.
See the section below for an example of Test driven development.
The Three Phases of Test Driven Development
Create Precise Tests: Developers must craft precise unit tests that validate specific features. These tests should compile but usually fail initially, based on initial assumptions.
Correct the Code: After a test fails, developers make minimal code changes to ensure it passes when re-executed.
Refactor the Code: Once a test passes, developers review the code for redundancy and optimization opportunities without altering external behavior.
Primarily, TDD takes up one of two approaches: Inside Out or Outside In.
In the Inside Out approach, the focus lies on the results or state.
Testing starts from the smallest unit level, and the architecture grows organically.
This typically involves minimal mocking and presents a way to prevent over-engineering.
The design happens during the refactor stage.
Conversely, the Outside In approach emphasizes user behavior.
Testing commences at the outermost level and moves inward with the evolving details.
This method heavily relies on mocking and stubbing external dependencies and aligns the code with the primary business needs.
The design occurs during the red stage.
The choice between these approaches relies on the specific situation.
Complex applications with numerous rapidly changing external dependencies, such as microservices, often align with the Outside In approach.
Conversely, smaller, monolithic applications are better suited for the Inside Out approach.
The Outside In approach also tends to complement front-end applications given their proximity to the end-user.
Benefits of Test Driven Development (TDD)
Various frameworks support TDD in different programming languages:
Effective TDD practices include:
The development team at a tech startup is tasked with creating a new fitness tracking app.
The app's goal is to allow users to track their daily physical activities, such as steps taken, calories burned, and workout routines, as well as monitor their progress over time.
The app will also offer motivational features, like setting personal goals and receiving awards for achieving them.
The team decides to use Test Driven Development (TDD) to ensure that the app is robust, easy to maintain, and flexible enough to handle future feature additions.
Using TDD will help them validate the functionality at every step and avoid future bugs as new features are added.
For this JavaScript project, the team chooses Jest, a popular testing framework that works well with applications developed with React, a library chosen for building the user interface.
npm install --save-dev jest
They set up Jest in their `package.json`` to automate testing processes and ensure integration with their continuous integration (CI) pipeline.
The development of the fitness tracking app is broken down into several features, starting with user authentication.
// auth.test.js
const auth = require('./auth')
test('User logs in using email and password', () => {
const user = auth.login('user@example.com', 'password123')
expect(user).toBe('Logged in successfully')
})
// auth.js
function login(email, password) {
// A simple check for example purposes
if (email === 'user@example.com' && password === 'password123') {
return 'Logged in successfully'
}
}
module.exports = { login }
After getting the green light from the test, the team refines the code to handle more complex scenarios like error handling, password encryption, and integration with their database.
Each new feature, such as tracking steps, logging workouts, and setting goals, undergoes the same TDD cycle.
The team writes tests first to define how the features should work, then writes the code to fulfill those tests, and finally refactors to optimize performance and maintainability.
The fitness tracking app was successfully developed and launched with high stability and minimal bugs at release.
The TDD approach significantly reduced the number of bugs found in production and improved the team's confidence in adding new features.
As development cycles become shorter and the demand for quality increases, TDD is set to become even more integral to software development processes.
It promotes a disciplined approach to coding and testing that aligns well with future technological advancements and methodologies.
What is the primary goal of Test-Driven Development (TDD)?
How does Test-Driven Development (TDD) differ from Behavior-Driven Development (BDD)?
What are the key benefits of implementing Test-Driven Development (TDD)?
How does Test-Driven Development (TDD) contribute to the Agile development approach?
Why is Test-Driven Development (TDD) considered a best practice in software development?
How can Test-Driven Development (TDD) benefit businesses and project management?
Are there different variations or types of Test-Driven Development (TDD)?